I recently implemented a swift generic input dialog for PyQt5 which extends the functionality of QInputDialog
. It supports multiple inputs in the same window, with text, numeric, boolean, and option inputs.
Its usage is simple and intuitive as demonstrated by the following code snippet:
import sys from PyQt5.QtWidgets import QApplication import GenericInputDialog app = QApplication(sys.argv) app.setQuitOnLastWindowClosed(True) accepted, values = GenericInputDialog.show_dialog("Test", [GenericInputDialog.TextLineInput('Text input'), GenericInputDialog.IntSpinInput('My Int', 10, -100, 100), GenericInputDialog.FloatSpinInput('My Float'), GenericInputDialog.IntSliderInput('My slider'), GenericInputDialog.BooleanInput('Bool value'), GenericInputDialog.OptionInput('My string options', [ 'option 1', 'option 2', 'option 3' ], 'option 3'), GenericInputDialog.OptionInput('My int options', [ ('option 1', 1.1), ('option 2', 2.2), ('option 3', 3.3) ], 2.2) ]) # Note: for option inputs, the value list can be a list # of strings, and then the output is the string itself, or a # list of tuples, where the first element is a string (the label) # and the second is the returned value (any). # The default value for options can be the label string, the # default returned value, or an integer index. # returned values can be accessed by key or by position print(values['My Int']) print(values[2]) # they can be iterated like a list for v in values: print(v)
Which produces the following:
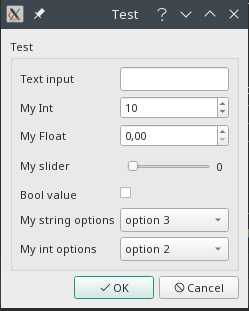
The code is in the public domain and is available at https://gist.github.com/fsantini/13bca4e5f57af1f5df66a3006ac441a9